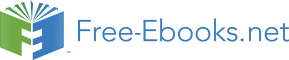

The Microsoft ADO.NET Entity Framework is an Object/Relational Mapping (ORM)
framework that enables developers to work with relational data as domain-specific
objects, eliminating the need for most of the data access plumbing code that
developers usually need to write.
Using the Entity Framework, developers issue queries using LINQ, then retrieve and
manipulate data as strongly typed objects. The Entity Framework’s ORM
implementation provides services like change tracking, identity resolution, lazy
loading, and query translation so that developers can focus on their application-
specific business logic rather than the data access fundamentals.
- Definition of Entity Framework given by Microsoft
www.ManzoorTheTrainer.com
6
Entity Framework - Simplified
Various approaches to work with Entity Framework:
Database First (This eBook is Focused on):
o Creating the model from database (Generating Classes or Class Diagram
from Database Tables).
Model First
o Creating the database from model (Generating Database Tables from Classes
or Class Diagram).
Code First (Some Time POCO)
o Coding classes against the existing Database is Code First.
o Creating the database from the coded classes(No class diagrams) is POCO.
www.ManzoorTheTrainer.com
7
Entity Framework - Simplified
Chapter 2: Creating an Entity Data Model
In this chapter, I’m going to show you How to create your Entity Data Model.
This is very simple. I’ve a database called as organization in which I’ve department
table and employee table.
I’m going to use the same database throughout our course on Entity Framework.
So, In Department table I’ve Did(Department ID), Dname(Department Name), HOD
and Gender in which Did is primary key.
In Employee table I’ve Eid(Employee ID), Ename(Employee Name), Esal(Employee
Salary), EGen(Employee Gender), EDOB(Employee Date Of Birth) and Did is the
foreign key from department table.
www.ManzoorTheTrainer.com
8
Entity Framework - Simplified
Creating Entity Data Model:
Create a web Project say EntityFrameworkPart-1
Lets create our entity data model(EDM) which is the heart of Entity Framework and it
is very simple.
I’ll goto my Project what I’ve created as EntityFrameworkPart-1
Right click the project
Add new item
Select ADO.NET Entity Data Model
And I’ll name it as OrganizationModel.edmx
Click Add
It is going to add in App_Code folder
I’ll say yes
It’ll fire a window with two options for me.
www.ManzoorTheTrainer.com
9
Entity Framework - Simplified
1. Generate Empty Database (Create a model from an existing database)
2. Empty Model (Create model first then create the database)
As I want to generate Entity Data Model from an existing database. Select Generate
Empty Database click Next.
You’ll see the database is organization and Entity connection string (same as your
connection string which provides the information about the provider, DataSource,
Initial Catalog , UserID, Password, Integrated Security….)
www.ManzoorTheTrainer.com
10
Entity Framework - Simplified
The connection string also contains some extra information as metadata about your
Entity Framework. Save entity connection settings in Web.Config as
OrganizationEntities click next.
At this point It’ll retrieve the database information (tables, stored procedures..) As
of now I’m going to work with two tables “tbl_Dept” and “tbl_Emp”. I’m going to add
these two tables to my Entity Data Model
Leave the options checked for Pluralize or Singularize generated object names and
Include foreign key columns in the model click on Finish.
Here’s my Entity Data Model
As you might have seen, in a single department I can have n number of employees.
www.ManzoorTheTrainer.com
11
Entity Framework - Simplified
So, we’ve one-to-many relationship and all the columns that I’ve in the database
are turned into properties for both the tables.
There are two extra properties called as Navigation Properties. That means whenever
I’ve relation between two tables for navigation from either tbl_Dept to tbl_Emp or
tbl_Emp to tbl_Dept .
So, this is my Entity Data Model.
Right click on Entity Data Model
Open with XML Editor Click Ok then Yes for Prompt window
This is the XML file that is generated automatical y for Entity Data Model
Let us have a look into this XML file and let us see what it contains
1. Runtime tag <edmx:Runtime> and
2. Designer tag <Designer>
www.ManzoorTheTrainer.com
12
Entity Framework - Simplified
The Designer tag contains some UI related to our Entity Data Model (Graphical table
data)
The Runtime tag consists of 3 parts
1. SSDL (Storage Schema Definition Language) [Info about database, tables,
columns.. for SQL data]
<edmx:StorageModels>
<Schema Namespace="OrganizationModel.Store " Alias="Self" Provider="System.Data.SqlClient "
ProviderManifestToken="2008"
xmlns:store="http://schemas.microsoft.com/ado/2007/12/edm/EntityStoreSchemaGenerator"
xmlns="http://schemas.microsoft.com/ado/2009/02/edm/ssdl">
<EntityContainer Name="OrganizationModelStoreContainer">
<EntitySet Name="tbl_Dept" EntityType="OrganizationModel.Store.tbl_Dept"
store:Type="Tables" Schema="dbo" />
<EntitySet Name="tbl_Emp" EntityType="OrganizationModel.Store.tbl_Emp" store:Type="Tables"
Schema="dbo" />
<AssociationSet Name="FK_tbl_Emp_tbl_Dept "
Association="OrganizationModel.Store.FK_tbl_Emp_tbl_Dept">
<End Role="tbl_Dept " EntitySet="tbl_Dept" />
<End Role="tbl_Emp" EntitySet="tbl_Emp" />
</AssociationSet>
</EntityContainer>
<EntityType Name="tbl_Dept">
<Key>
<PropertyRef Name="Did" />
</Key>
<Property Name="Did" Type="int" Nul able="false" StoreGeneratedPattern="Identity" />
<Property Name="Dname" Type="varchar" MaxLength="50" />
<Property Name="HOD" Type="varchar" Nul able="false" MaxLength="50" />
<Property Name="Gender" Type="varchar" Nul able="false" MaxLength="50" />
<Property Name="Active" Type="bit" />
</EntityType>
<EntityType Name="tbl_Emp">
<Key>
<PropertyRef Name="Eid" />
</Key>
<Property Name="Eid" Type="int" Nul able="false" StoreGeneratedPattern="Identity" />
<Property Name="EName" Type="varchar" Nul able="false" MaxLength="50" />
<Property Name="ESal" Type="float" Nul able="false" />
<Property Name="EGen" Type="varchar" Nul able="false" MaxLength="10" />
<Property Name="EDOB" Type="datetime" Nul able="false" />
<Property Name="Did" Type="int" />
</EntityType>
<Association Name="FK_tbl_Emp_tbl_Dept">
<End Role="tbl_Dept" Type="OrganizationModel.Store.tbl_Dept" Multiplicity="0..1" />
<End Role="tbl_Emp" Type="OrganizationModel.Store.tbl_Emp" Multiplicity="*" />
<ReferentialConstraint >
<Principal Role="tbl_Dept">
<PropertyRef Name="Did" />
</Principal>
<Dependent Role="tbl_Emp">
<PropertyRef Name="Did" />
</Dependent>
</ReferentialConstraint >
</Association>
<Function Name="SP_GetAllEmployee" Aggregate="false" BuiltIn="false"
NiladicFunction="false" IsComposable="false"
ParameterTypeSemantics="AllowImplicitConversion" Schema="dbo" />
<Function Name="SP_GetEmployeesByDid" Aggregate="false" BuiltIn="false" NiladicFunction="false"
IsComposable="false" ParameterTypeSemantics="AllowImplicitConversion" Schema="dbo">
<Parameter Name="Did" Type="int" Mode="In" />
</Function>
www.ManzoorTheTrainer.com
13
Entity Framework - Simplified
<Function Name="SP_GetEmpNameAndSalaryByEid" Aggregate="false" BuiltIn="false"
NiladicFunction="false" IsComposable="false"
ParameterTypeSemantics="AllowImplicitConversion" Schema="dbo">
<Parameter Name="Eid" Type="int" Mode="In" />
<Parameter Name="EName" Type="varchar" Mode="InOut" />
<Parameter Name="ESal" Type="float" Mode="InOut" />
</Function>
</Schema></edmx:StorageModels>
2. CSDL (Conceptual Schema Definition Language) [Properties for each table and each
column.. for C# data]
<edmx:ConceptualModels >
<Schema Namespace="OrganizationModel" Alias="Self"
xmlns:annotation="http://schemas.microsoft.com/ado/2009/02/edm/annotation"
xmlns="http://schemas.microsoft.com/ado/2008/09/edm">
<EntityContainer Name="OrganizationEntities" annotation:LazyLoadingEnabled="true">
<EntitySet Name="tbl_Dept" EntityType="OrganizationModel.tbl_Dept" />
<EntitySet Name="tbl_Emp" EntityType="OrganizationModel.tbl_Emp" />
<AssociationSet Name="FK_tbl_Emp_tbl_Dept"
Association="OrganizationModel.FK_tbl_Emp_tbl_Dept">
<End Role="tbl_Dept " EntitySet="tbl_Dept" />
<End Role="tbl_Emp" EntitySet="tbl_Emp" />
</AssociationSet>
<FunctionImport Name="SP_GetAllEmployee"
ReturnType="Collection(OrganizationModel.SP_GetAl Employee_Result)" />
<FunctionImport Name="SP_GetEmployeesByDid"
ReturnType="Collection(OrganizationModel.SP_GetEmployeesByDid_Result)">
<Parameter Name="Did" Mode="In" Type="Int32" />
</FunctionImport >
<FunctionImport Name="SP_GetEmpNameAndSalaryByEid">
<Parameter Name="Eid" Mode="In" Type="Int32" />
<Parameter Name="EName" Mode="InOut " Type="String" />
<Parameter Name="ESal" Mode="InOut" Type="Double" />
</FunctionImport >
</EntityContainer>
<EntityType Name="tbl_Dept">
<Key>
<PropertyRef Name="Did" />
</Key>
<Property Name="Did" Type="Int32" Nul able="false"
annotation:StoreGeneratedPattern="Identity" />
<Property Name="Dname" Type="String" MaxLength="50" Unicode="false" FixedLength="false" />
<Property Name="HOD" Type="String" Nul able="false" MaxLength="50" Unicode="false"
FixedLength="false" />
<Property Name="Gender" Type="String" Nul able="false" MaxLength="50" Unicode="false"
FixedLength="false" />
<NavigationProperty Name="tbl_Emp" Relationship="OrganizationModel.FK_tbl_Emp_tbl_Dept"
FromRole="tbl_Dept" ToRole="tbl_Emp" />
<Property Type="Boolean" Name="Active" />
</EntityType>
<EntityType Name="tbl_Emp">
<Key>
<PropertyRef Name="Eid" />
</Key>
<Property Name="Eid" Type="Int32" Nul able="false"
annotation:StoreGeneratedPattern="Identity" />
<Property Name="EName" Type="String" Nul able="false" MaxLength="50" Unicode="false"
FixedLength="false" />
<Property Name="ESal" Type="Double" Nul able="false" />
<Property Name="EGen" Type="String" Nul able="false" MaxLength="10" Unicode="false"
FixedLength="false" />
<Property Name="EDOB" Type="DateTime" Nul able="false" />
<Property Name="Did" Type="Int32" />
www.ManzoorTheTrainer.com
14
Entity Framework - Simplified
<NavigationProperty Name="tbl_Dept"
Relationship="OrganizationModel.FK_tbl_Emp_tbl_Dept " FromRole="tbl_Emp" ToRole="tbl_Dept "
/>
</EntityType>
<Association Name="FK_tbl_Emp_tbl_Dept">
<End Role="tbl_Dept" Type="OrganizationModel.tbl_Dept" Multiplicity="0..1" />
<End Role="tbl_Emp" Type="OrganizationModel.tbl_Emp" Multiplicity="*" />
<ReferentialConstraint >
<Principal Role="tbl_Dept">
<PropertyRef Name="Did" />
</Principal>
<Dependent Role="tbl_Emp">
<PropertyRef Name="Did" />
</Dependent>
</ReferentialConstraint >
</Association>
<ComplexType Name="SP_GetAl Employee_Result ">
<Property Type="Int32" Name="Eid" Nul able="false" />
<Property Type="String" Name="EName" Nul able="false" MaxLength="50" />
<Property Type="Double" Name="Esal" Nul able="false" />
<Property Type="DateTime" Name="EDOB" Nul able="false" Precision="23" />
<Property Type="String" Name="Dname" Nul able="true" MaxLength="50" />
<Property Type="String" Name="HOD" Nul able="false" MaxLength="50" />
</ComplexType>
<ComplexType Name="SP_GetEmployeesByDid_Result">
<Property Type="Int32" Name="Eid" Nul able="false" />
<Property Type="String" Name="EName" Nul able="false" MaxLength="50" />
<Property Type="Double" Name="ESal" Nul able="false" />
<Property Type="String" Name="EGen" Nul able="false" MaxLength="10" />
<Property Type="DateTime" Name="EDOB" Nul able="false" Precision="23" />
<Property Type="Int32" Name="Did" Nul able="true" />
</ComplexType>
</Schema>
</edmx:ConceptualModels >
3. C-S Mapping [Column Mappings]
<edmx:Mappings>
<Mapping Space="C-S" xmlns="http://schemas.microsoft.com/ado/2008/09/mapping/cs">
<EntityContainerMapping StorageEntityContainer="OrganizationModelStoreContainer"
CdmEntityContainer="OrganizationEntities">
<EntitySetMapping Name="tbl_Dept "><EntityTypeMapping
TypeName="OrganizationModel.tbl_Dept"><MappingFragment StoreEntitySet="tbl_Dept">
<ScalarProperty Name="Active" ColumnName="Active" />
<ScalarProperty Name="Did" ColumnName="Did" />
<ScalarProperty Name="Dname" ColumnName="Dname" />
<ScalarProperty Name="HOD" ColumnName="HOD" />
<ScalarProperty Name="Gender" ColumnName="Gender" />
</MappingFragment ></EntityTypeMapping></EntitySetMapping>
<EntitySetMapping Name="tbl_Emp"><EntityTypeMapping
TypeName="OrganizationModel.tbl_Emp"><MappingFragment StoreEntitySet="tbl_Emp">
<ScalarProperty Name="Eid" ColumnName="Eid" />
<ScalarProperty Name="EName" ColumnName="EName" />
<ScalarProperty Name="ESal" ColumnName="ESal" />
<ScalarProperty Name="EGen" ColumnName="EGen" />
<ScalarProperty Name="EDOB" ColumnName="EDOB" />
<ScalarProperty Name="Did" ColumnName="Did" />
</MappingFragment ></EntityTypeMapping></EntitySetMapping>
<FunctionImportMapping FunctionImportName="SP_GetAllEmployee"
FunctionName="OrganizationModel.Store.SP_GetAl Employee ">
<ResultMapping>
<ComplexTypeMapping TypeName="OrganizationModel.SP_GetAl Employee_Result ">
<ScalarProperty Name="Eid" ColumnName="Eid" />
<ScalarProperty Name="EName" ColumnName="EName" />
<ScalarProperty Name="Esal" ColumnName="Esal" />
<ScalarProperty Name="EDOB" ColumnName="EDOB" />
www.ManzoorTheTrainer.com
15
Entity Framework - Simplified
<ScalarProperty Name="Dname" ColumnName="Dname" />
<ScalarProperty Name="HOD" ColumnName="HOD" />
</ComplexTypeMapping>
</ResultMapping>
</FunctionImportMapping>
<FunctionImportMapping FunctionImportName="SP_GetEmployeesByDid"
FunctionName="OrganizationModel.Store.SP_GetEmployeesByDid ">
<ResultMapping>
<ComplexTypeMapping TypeName="OrganizationModel.SP_GetEmployeesByDid_Result ">
<ScalarProperty Name="Eid" ColumnName="Eid" />
<ScalarProperty Name="EName" ColumnName="EName" />
<ScalarProperty Name="ESal" ColumnName="ESal" />
<ScalarProperty Name="EGen" ColumnName="EGen" />
<ScalarProperty Name="EDOB" ColumnName="EDOB" />
<ScalarProperty Name="Did" ColumnName="Did" />
</ComplexTypeMapping>
</ResultMapping>
</FunctionImportMapping>
<FunctionImportMapping FunctionImportName="SP_GetEmpNameAndSalaryByEid"
FunctionName="OrganizationModel.Store.SP_GetEmpNameAndSalaryByEid " />
</EntityContainerMapping>
</Mapping>
</edmx:Mappings>
CLR generates 3 different files for Runtime tag at runtime.
www.ManzoorTheTrainer.com
16
Entity Framework - Simplified