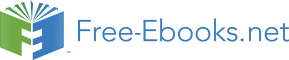

ASP.NET Code Block Types
Interaction with the page from these blocks is limited, since the code is executed during the render phase of the page life cycle
<% Trace.Warn("Embedded Code Block", "Hello World"); %>
Let’s have a look at the different types of syntax we can use for code blocks in ASP.NET pages. There are really four types of code blocks, and the first one is different from the others:
First of all we have ASP.NET expressions which look like <%$ AppSettings:Key %> <asp:Label runat="server" Text="<%$ AppSettings:Key %>" />
ASP.NET DataBinding syntax
The next code construct is the data-binding syntax: <%# Eval("Value") %> which is used to bind to properties to data on demand.
Statement and Expression/Evaluated Code Blocks Display some values
<%
string message = "Hello World!";
Response.Write(message);
%>
These are delimited by <%= and %> and the content of this code block becomes the parameter to the HtmlTextWrite.Write() method. Therefore, the code inside this type of code block should be an expression, and not a statement.
<%= String.Format("The title of this page is: {0}", this.Title ?? "n/a") %>
What method do you use to explicitly kill a users session?
Session.Abandon
Which two properties are on every validation control?
1. ControlToValidate
2. ErrorMessage
What are the validation controls in asp.net?
There are 5 validation controls in asp.net
1. RequiredFieldValidator
2. RangeValidator
3. RegularExpressionValidator
4. CompareValidator
5. CustomValidator
ValidationSummary is not a validation control but a control that displays summary of all error occurs while validating the page.
How to load a user control dynamically in runtime?
Control c = (Control)Page.LoadControl("~/usercontrol/MyUserControl.ascx"); Page.Form.Controls.Add(c);
How to get the authentication mode from web.config file programmatically at runtime?
System.Web.Configuration.AuthenticationSection section =
(AuthenticationSection)WebConfigurationManager.GetSection("system.web/authentication"); Label1.Text = section.Mode.ToString();
What’s the difference between Response.Write() and Response.Output.Write()?
Response.Output.Write() allows you to write formatted output.
What’s a bubbled event?
When you have a complex control, like DataGrid, writing an event processing routine for each object (cell, button, row, etc.) is quite tedious. The controls can bubble up their event handlers, allowing the main DataGrid event handler to take care of its constituents.
ASP.NET Compilation Tool (Aspnet_compiler.exe)
The ASP.NET Compilation tool (Aspnet_compiler.exe) enables you to compile an ASP.NET Web application, either in place or for deployment to a target location such as a production server. In-place compilation helps application performance because end users do not encounter a delay on the first request to the application while the application is compiled.
Compilation for deployment can be performed in one of two ways: one that removes all source files, such as code-behind and markup files, or one that retains the markup files.
What is different between WebUserControl and in WebCustomControl?
Web user controls :- Web User Control is Easier to create and another thing is that its support is limited for users who use a visual design tool one good thing is that its contains static layout one more thing a separate copy is required for each application.
Web custom controls: - Web Custom Control is typical to create and good for dynamic layout and another thing is that it has full tool support for user and a single copy of control is required because it is placed in Global Assembly cache.
What is smart navigation?
Enable smart navigation by using the Page.SmartNavigation property. When you set the Page.SmartNavigation property to true, the following smart navigation features are enabled:
Note: Smart navigation is deprecated in Microsoft ASP.NET 2.0 and is no longer supported by Microsoft Product Support Services
How many types of cookies are there in ASP.NET ?
If you create a cookie without specifying an expiration date, you are creating an in-memory cookie, which lives for that browser session only. The following illustrates the script that would be used for an in-memory cookie:
Response.Cookies("SiteArea") = "TechNet"
The following illustrates the script used to create a persistent cookie:
Response.Cookies("SiteArea") = "TechNet"
Response.Cookies("SiteArea").Expires = "August 15, 2000"
TODO List
Explain how PostBacks work, on both the client-side and server-side. How do I chain my own JavaScript? How does ViewState work and why is it either useful or evil?
What is the OO relationship between an ASPX page and its CS/VB code behind file in ASP.NET 1.1? In 2.0?
What happens from the point an HTTP request is received on a TCP/IP port up until the Page fires the On_Load event?
How does IIS communicate at runtime with ASP.NET? Where is ASP.NET at runtime in IIS5? IIS6?
What is an assembly binding redirect? Where are the places an administrator or developer can affect how assembly binding policy is applied?
Compare and contrast LoadLibrary(), CoCreateInstance(), CreateObject() and Assembly.Load().