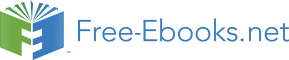

3.1. Using the python interpreter prompt .......................................................................... 6
3.2. Using a Source File ............................................................................................... 7
4.1. Using Variables and Literal constants ...................................................................... 14
5.1. Using Expressions ............................................................................................... 22
6.1. Using the if statement .......................................................................................... 24
6.2. Using the while statement ..................................................................................... 26
6.3. Using the for statement ......................................................................................... 27
6.4. Using the break statement ..................................................................................... 29
6.5. Using the continue statement ................................................................................. 30
7.1. Defining a function .............................................................................................. 32
7.2. Using Function Parameters .................................................................................... 33
7.3. Using Local Variables .......................................................................................... 34
7.4. Using the global statement .................................................................................... 35
7.5. Using Default Argument Values ............................................................................. 36
7.6. Using Keyword Arguments ................................................................................... 37
7.7. Using the literal statement ..................................................................................... 38
7.8. Using DocStrings ................................................................................................ 39
8.1. Using the sys module ........................................................................................... 41
8.2. Using a module's __name__ .................................................................................. 43
8.3. How to create your own module ............................................................................. 43
8.4. Using the dir function .......................................................................................... 45
9.1. Using lists .......................................................................................................... 47
9.2. Using Tuples ...................................................................................................... 49
9.3. Output using tuples .............................................................................................. 50
9.4. Using dictionaries ............................................................................................... 51
9.5. Using Sequences ................................................................................................. 53
9.6. Objects and References ........................................................................................ 55
9.7. String Methods ................................................................................................... 56
10.1. Backup Script - The First Version ......................................................................... 58
10.2. Backup Script - The Second Version ..................................................................... 60
10.3. Backup Script - The Third Version (does not work!) ................................................. 62
10.4. Backup Script - The Fourth Version ...................................................................... 63
11.1. Creating a Class ................................................................................................ 68
11.2. Using Object Methods ........................................................................................ 69
11.3. Using the __init__ method ................................................................................... 69
11.4. Using Class and Object Variables ......................................................................... 71
11.5. Using Inheritance .............................................................................................. 73
12.1. Using files ........................................................................................................ 76
12.2. Pickling and Unpickling ...................................................................................... 77
13.1. Handling Exceptions .......................................................................................... 80
13.2. How to Raise Exceptions .................................................................................... 81
13.3. Using Finally .................................................................................................... 82
14.1. Using sys.argv .................................................................................................. 84
15.1. Using List Comprehensions ................................................................................. 89
15.2. Using Lambda Forms ......................................................................................... 90