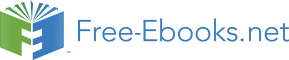

Design Pattern
Command
Encapsulate a request as an object, thereby letting you parameterize clients with different requests, queue or log requests, and support undoable operations.
/*the Command interface*/
public interface Command {
void execute();
}
/*the Invoker class*/
import java.util.List;
import java.util.ArrayList;
public class Switch {
private List<Command> history = new ArrayList<Command>();
public Switch () {
}
public void storeAndExecute(Command cmd) {
this .history.add(cmd); // optional
cmd.execute();
}
}
/*the Receiver class*/
public class Light {
public Light() {
}
public void turnOn() {
System.out.println("The light is on");
}
public void turnOff() {
System.out.println("The light is off");
}
}
/*the Command for turning off the light - ConcreteCommand #2*/
public class FlipDownCommand implements Command {
private Light theLight;
public FlipDownCommand(Light light) {
this .theLight = light;
}
public void execute() {
theLight.turnOff();
}
}
/*The test class or client*/
public class PressSwitch {
public static void main(String[] args){
Light lamp = new Light();
Command switchUp = new FlipUpCommand(lamp);
Command switchDown = new FlipDownCommand(lamp);
Switch s = new Switch();
try {
If (args[0].equalsIgnoreCase("ON")) {
s.storeAndExecute(switchUp);
System.exit(0);
}
If (args[0].equalsIgnoreCase("OFF")) {
s.storeAndExecute(switchDown);
System.exit(0);
}
System.out.println("Argument \" ON \" or \" OFF \" is required.");
}
catch (Exception e) {
System.out.println("Argument's required.");
}
}
}
Iterator
Provide a way to access the elements of an aggregate object sequentially without exposing its underlying representation.
Null object
Avoid null references by providing a default object.
/* Null Object Pattern implementation:
*/
using System;
// Animal interface is the key to compatibility for Animal implementations below.
interface IAnimal
{
void MakeSound();
}
// Dog is a real animal.
class Dog : IAnimal
{
public void MakeSound()
{
Console.WriteLine("Woof!");
}
}
// The Null Case: this NullAnimal class should be instantiated and used in place of C# null keyword.
class NullAnimal : IAnimal
{
public void MakeSound()
{
// Purposefully provides no behaviour.
}
}
/* =========================
* Simplistic usage example in a Main entry point.
*/
static class Program
{
static void Main()
{
IAnimal dog = new Dog();
dog.MakeSound(); // outputs "Woof!"
/* Instead of using C# null, use a NullAnimal instance.
* This example is simplistic but conveys the idea that if a NullAnimal instance is used then the program
* will never experience a .NET System.NullReferenceException at runtime, unlike if C# null was used.
*/
IAnimal unknown = new NullAnimal(); //<< replaces: IAnimal unknown = null;
unknown.MakeSound(); // outputs nothing, but does not throw a runtime exception
}
}
Observer or Publish/subscribe
Define a one-to-many dependency between objects where a state change in one object results in all its dependents being notified and updated automatically.
// Observer pattern -- Structural example
using System;
using System.Collections.Generic;
namespace DoFactory.GangOfFour.Observer.Structural
{
/// <summary>
/// MainApp startup class for Structural
/// Observer Design Pattern.
/// </summary>
class MainApp
{
/// <summary>
/// Entry point into console application.
/// </summary>
static void Main()
{
// Configure Observer pattern
ConcreteSubject s = new ConcreteSubject();
s.Attach(new ConcreteObserver(s, "X"));
s.Attach(new ConcreteObserver(s, "Y"));
s.Attach(new ConcreteObserver(s, "Z"));
// Change subject and notify observers
s.SubjectState = "ABC";
s.Notify();
// Wait for user
Console.ReadKey();
}
}
/// <summary>
/// The 'Subject' abstract class
/// </summary>
abstract class Subject
{
private List<Observer> _observers = new List<Observer>();
public void Attach(Observer observer)
{
_observers.Add(observer);
}
public void Detach(Observer observer)
{
_observers.Remove(observer);
}
public void Notify()
{
foreach (Observer o in _observers)
{
o.Update();
}
}
}
/// <summary>
/// The 'ConcreteSubject' class
/// </summary>
class ConcreteSubject : Subject
{
private string _subjectState;
// Gets or sets subject state
public string SubjectState
{
get { return _subjectState; }
set { _subjectState = value; }
}
}
/// <summary>
/// The 'Observer' abstract class
/// </summary>
abstract class Observer
{
public abstract void Update();
}
/// <summary>
/// The 'ConcreteObserver' class
/// </summary>
class ConcreteObserver : Observer
{
private string _name;
private string _observerState;
private ConcreteSubject _subject;
// Constructor
public ConcreteObserver(
ConcreteSubject subject, string name)
{
this._subject = subject;
this._name = name;
}
public override void Update()
{
_observerState = _subject.SubjectState;
Console.WriteLine("Observer {0}'s new state is {1}",
_name, _observerState);
}
// Gets or sets subject
public ConcreteSubject Subject
{
get { return _subject; }
set { _subject = value; }
}
}
}
Visitor
Represent an operation to be performed on the elements of an object structure. Visitor lets you define a new operation without changing the classes of the elements on which it operates.
/ Visitor pattern -- Real World example
using System;
using System.Collections.Generic;
namespace DoFactory.GangOfFour.Visitor.RealWorld
{
/// <summary>
/// MainApp startup class for Real-World
/// Visitor Design Pattern.
/// </summary>
class MainApp
{
/// <summary>
/// Entry point into console application.
///